Top 50 Salesforce Developer Interview Questions and Answers for 2025
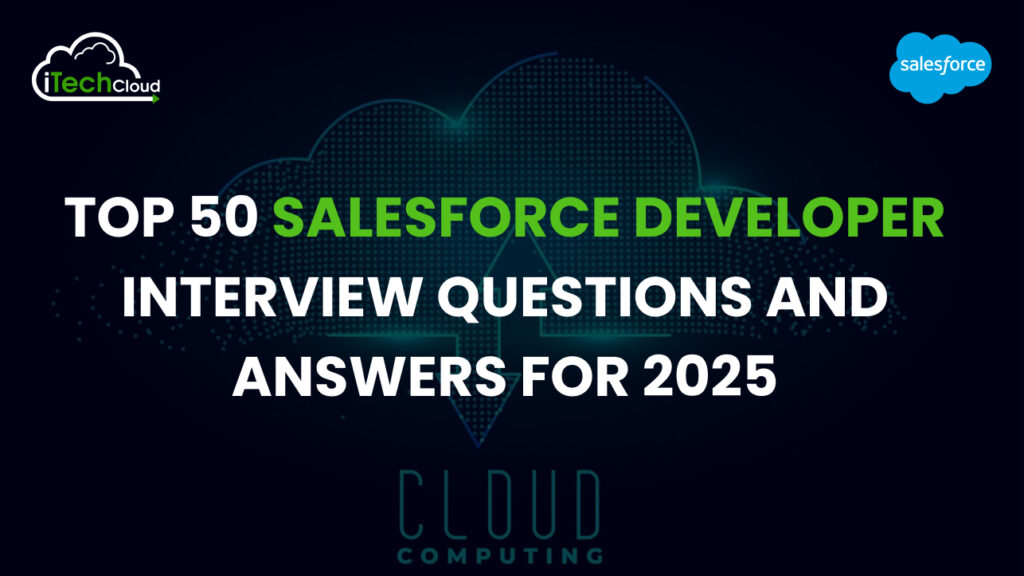
Here are the top 50 salesforce developer interview questions and answers for 2025:
Table of Contents
Basic Level
1. What is Apex?
Apex is Salesforce’s proprietary, strongly typed, object-oriented programming language that allows developers to execute flow and transaction control statements on Salesforce servers, in conjunction with calls to the API.
2. What is a trigger in Salesforce?
Triggers are Apex code that executes before or after specific data manipulation language (DML) events occur, such as insert, update, delete, or undelete. They allow developers to perform custom actions.
3. What’s the Difference Between a Trigger and a Workflow?
Triggers are code-based and allow complex logic across multiple objects. Workflows are point-and-click tools limited to single-object automations like field updates and email alerts.
4. What is SOQL?
SOQL (Salesforce Object Query Language) is used to retrieve records from a single object or related objects. It is similar to SQL but tailored for Salesforce data models.
5. What is SOSL?
SOSL (Salesforce Object Search Language) is used for searching text across multiple objects and fields simultaneously. It’s used for broader text searches.
6. What are governor limits?
Governor limits are runtime limits enforced by Salesforce to ensure shared resource availability. These include limits on SOQL queries, DML operations, CPU time, heap size, etc.
7. What is the MVC architecture in Salesforce?
MVC stands for Model (data), View (UI), and Controller (logic). Salesforce uses this pattern: Objects = Model, Visualforce/Lightning = View, and Apex = Controller.
8. What is a static resource?
Static resources allow developers to upload external files such as JavaScript, CSS, images, and ZIP files to reference in Visualforce or Lightning Components.
9. What is the @future annotation in Apex?
@future is used for running methods asynchronously in the background, especially for operations like callouts or large data processing that don’t require immediate results.
10. What are collections in Apex?
Collections in Apex are data structures to hold multiple records.
- List: Ordered collection (like arrays)
- Set: Unordered with no duplicates
- Map: Key-value pairs
🔹 Intermediate Level
11. Trigger vs. Process Builder: Which One to Use?
Use triggers for complex logic, bulk operations, or cross-object manipulations. Use Process Builder for simple, single-object automations.
12. Types of Triggers in Apex?
Triggers are either before or after and operate on insert, update, delete, and undelete.
13. What is Batch Apex?
Batch Apex handles large volumes of data (millions of records) by breaking them into manageable chunks and processing them asynchronously using the database. Batchable interface.
14. What is Queueable Apex?
Queueable Apex allows asynchronous processing with the ability to chain jobs and is more flexible than @future.
15. Difference Between Class and Interface?
A class is a blueprint with implementations. An interface is a contract containing method signatures only. Interfaces enforce structure without code.
16. Access Modifiers in Apex?
Apex uses private, public, protected, and global to define class/member visibility. Global allows access across namespaces.
17. What is a custom setting?
Custom settings allow storing configuration data at the org or user level. They are cached, making them faster than querying records.
18. With Sharing vs. Without Sharing in Apex?
Sharing enforces the user’s record-level permissions. without sharing, ignores them. Choose based on business logic.
19. Difference between insert and Database.insert?
Insert throws an exception and halts if any record fails. Database.insert allows partial success and provides a result object.
20. How to Call a Class in Apex?
Use static method calls (ClassName.methodName()) or create an instance of the class and call methods using dot notation.
🔹 Advanced Level
21. What is Custom Metadata Type?
Custom metadata types are similar to custom settings but are deployable and packageable, making them ideal for app-level config data.
22. What is Lightning Web Component (LWC)?
LWC is Salesforce’s modern JavaScript-based web component framework, providing a lightweight, performant way to build UI on the Lightning platform.
23. What is @AuraEnabled?
@AuraEnabled exposes Apex methods to Lightning components (Aura and LWC) for client-server communication.
24. What is a wrapper class in Apex?
A wrapper class is a custom data structure that wraps multiple fields or objects, often used in Visualforce pages or complex logic handling.
25. Trigger.new vs. Trigger.old
Trigger.new contains the updated/new values of records. Trigger.old contains the original values (used in update and delete contexts).
26. How to Prevent Trigger Recursion?
Use static variables (flags) in a helper class to prevent code from executing recursively during trigger calls.
27. Types of Relationships in Salesforce?
- Lookup: Loose relationship
- Master-Detail: Parent-child with dependency
- Many-to-Many: Implemented using a junction object
28. What is a test class?
Test classes validate Apex code logic and ensure stability during deployment. Salesforce requires at least 75% test coverage for deployment.
29. What is Schedule Apex?
Scheduled Apex uses the Schedulable interface and allows you to schedule classes to run at specific times using System. schedule().
30. What is Dynamic Apex?
Dynamic Apex allows code to adapt at runtime using features like Schema.describe, dynamic SOQL, and SObject field manipulation.
🔹 LWC & Frontend
31. How to Pass Data from Parent to Child in LWC?
Use the @api decorator on a property in the child component and bind the value from the parent.
32. What is the Wire Service?
The wire service in LWC fetches data reactively from Salesforce (e.g., Apex, UI APIs) without writing explicit logic.
33. How to Call Apex in LWC?
Import the method decorated with @AuraEnabled(cacheable=true/false) and invoke using @wire or imperatively.
34. Component Communication in LWC?
Use:
- @api: Parent to child
- Custom events: Child to parent
- Pub-sub or LMS: Unrelated components
35. What is Lightning Data Service (LDS)?
LDS allows you to perform CRUD operations without Apex and handles record sharing, field-level security, and cache management.
🔹 Deployment & Integration
36. Deployment Tools in Salesforce?
Salesforce supports deployment through:
- Change Sets (UI)
- ANT Migration Tool
- Salesforce CLI
- VS Code (SFDX)
37. Change Set vs. ANT?
Change sets are UI-based and only available between connected orgs. ANT allows scriptable, reusable deployments across any orgs.
38. How to Integrate Salesforce with External Systems?
Use REST/SOAP APIs, named credentials, external services, or platform events based on the use case.
39. What is a named credential?
A named credential simplifies authentication for outbound calls by storing endpoints and credentials securely.
40. What is a connected app?
Connected Apps integrate Salesforce with external applications using OAuth protocols for secure data access.
🔹 Scenario-Based
41. How to Handle Large Data Volume in Triggers?
Use bulk-safe logic, avoid SOQL/DML in loops, use collections, and consider async processing like Batch or Queueable.
42. Best Practices for Writing Triggers?
- One trigger per object
- Use handler class.
- Bulkify logic
- Handle recursion
- Write proper test cases.
43. How to Ensure Test Data Isolation?
Use @testSetup methods to create test data. Avoid using SeeAllData=true unless absolutely necessary.
44. Can You Make a Callout in a Trigger?
No. Triggers are synchronous and disallow callouts. Use asynchronous methods like @future or Queueable.
45. Difference Between REST API and SOAP API?
- REST: Lightweight, uses JSON, great for mobile
- SOAP: XML-based, supports more complex standards
🔹 Miscellaneous
46. What is a platform event?
Platform Events enable event-driven architecture in Salesforce, allowing systems to communicate via publish/subscribe.
47. What are Flow and Flow Builder?
Flow is a tool for automating complex business processes. Flow Builder is its visual design interface.
48. Why Avoid DML in a Loop?
Causes governor limit exceptions. Instead, gather records in a list and perform a single DML outside the loop.
49. How to Monitor Apex Execution?
Use debug logs, the developer console, the Apex execution log, and performance monitoring tools.
50. What’s New in Spring ’25 for Developers?
Spring ‘25 introduces features like improved LWC APIs, AI-powered Apex suggestions, advanced Flow elements, and enhanced deployment tools.